Hello everyone! It's cole7778 here today with a cool new tutorial on General Scripting!
Today we have a special Guest, Her name is Weeve! here's a picture of her below.
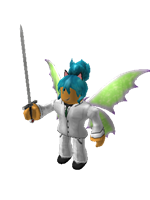
Ok, so, first off, you need to know what a variable is,
A variable is merely a value holder, it is created by
variablename = variablevalue
so,
cat = 5
now, you can also modify the value of a variable,
cat = 5
cat = cat + 1
cat would now be 6, the other mathematical marks you can use are (*) which is times, (/) which is divided by, (-) which is minus, (^) for advanced math, you will almost never use ^
next, you need to learn about the OUTPUT, if you dont use Roblox Studio, I highly suggest it, it provides a way better scripting atmosphere,
you can open up output on roblox studio by View>Output, I suggest the position to be bottom of screen, about 1/5 of your screen size, keep it pinned, if it is unpinned, click the little pin symbol on its window.
what the output is used for is error checking, if you see red words, that means that a script broke, if you see yellow words, thats roblox talking to you, grey words are printed words
to print a word, simple use print()
wait(1) -- gives time to load, waits one second
cat = 5
cat = cat + 1
print(cat)
now this little script should paste to the output "6", you can effectively use printing to locate errors by making it print before where you suspect the error is, and one after it, so, if both print, the error is after you suspected, if none print, the error is before, and if one prints, the error is on that line,
now you should learn about loops, I <3 loops.
while cat <= 10 do
wait(1) -- gives time to load, waits one second
cat = 5
cat = cat + 1
print(cat)
end
I introduced two new things now, one being the while loop, one being "<" and "="
the while loop simply keeps running over and over again until whats between "while" and "do" dosent fit your statement. ALWAYS, I repeat ALWAYS have a wait in your while loop, or you will crash your computer if something goes wrong (happened many times to me.. its a bran killer to lose 3 hours progress, and 300 lines..) also, if you want the loop to be infinite, simple put "while true do", infinite loops are very common.
the "<" sign mean "smaller than" and the "=" sign means is equal to, but if you want to check if two things are equal, you have to put two equal signs "==" because, its comparing both sides, your script will say "unexpected symbol at line.." and crash if you dont, you can also use "<=" and ">=" and ">" and "<" and "~="(not equal to) and "=="
lets make something useful :)
turnacuteness = 20
while true do
wait(0.1) -- gives time to load, waits one second
turn = CFrame.Angles(0, math.pi/turnacuteness, 0)
script.Parent.CFrame = script.Parent.CFrame * turn
end
put a brick in workspace, and this script inside of it, what this script does is constantly CFrames a part :) it just looks cool..
for more info, see (http://wiki.roblox.com/index.php/CFrame)
Ok, next, the for loop, a loop I have recently come to appreciate and respect, basically, it runs for as long as you tell it to, and is useful in breaking apart tables and editing their contents, and example of a for loop being:
cat = 5
for i=1,cat do
cat = cat-1
end
this loop runs "cat" times, then stops, I used this to gradually lower cat to "0", basically, i is a new variable, its value is the loop its on, so, the first time it ran, i is 1, the second time, i is 2, etc, the 1,cat part tells it to run from "1" until it reaches "cat", in this case, it runs five times, you dont have to use a variable, you could just say "for i=1,5 do", and it dosent have to start at "1", but starting at 1 will be very commonly used, an example of making use of i being
for i=1,20 do
wait(0.1)
script.Name = "I'v ran "..i.." loops, up hill, both ways"
end
this example makes use of i, in which, the script changes its name, I have also introduced "..", ".." merely switches the text from string mode to variable mode, its useful in many cases, :)
next, is a table, its another type of variable, you make one by:
cat = {1,3,3,7}
this is an example of a table holding four values, you can access them by:
cat = {1,3,3,7}
print(cat[4])
that would print the fourth value in the table, or "7", you can also add to a table,
cat = {1,3,3,7}
cat[5] = " pwns all"
print(cat[5])
in this example, I added a text value to it :) although, one downside of a table is, you cant print the whole thing, unless you use a for loop, watch:
cat = {1,3,3,7," pwns all"}
compiledstring = ""
for i=1,#cat do
compiledstring = compiledstring..cat[i]
end
print(compiledstring)
I introduced the "#" sign to you, basically, it gets the number of values in a table, what this script will print is "1337 pwns all", neat, eh? did you catch how I did it? I added to the string value by value from the table, again making use of "i"
another function you can use is pretty neat:
c = game.Workspace:GetChildren()
for i=1,#c do
c[i].Name = "Watch out! a scripter got a hold of Workspace!"
end
like, no? it renames everything in workspace to the string, if you wanted to do something evil, here is a time to make use of the remove() function :3, the GetChildren() function just compiles a table of all the instances in Workspace
ok, next, player made functions, they are very useful indeed, they help organize monotonous amounts of code, or simple help you with making a chain reaction
function plusplus(number)
number = number + 1
return number
end
myfirstreturn = plusplus(1337)
hmm, you should understand this pretty well by now, but, the "function plusplus()" part makes a new function, and the "number" inside the parentheses is what you can give it, it accepts mroe than one number, and will accept any other type of variable as well :), to run a function, you simply say the function name and parentheses, and any number you want to give it, "plusplus(5)", you dont even need the "variable =" part, unless you want to return something, a return basically send back info to the function call(plusplus() is called a function call), you can access it by using the variable = plusplus(189) :)
Oh.. and im sure you want something neat to do now, dont you..
Go make it! PM me the link to your model, your turn, lazy XD
Thanks for reading! I hope this was a big help to you all. Want to have a specific tutorial? Message me on roblox explaining what you want a tutorial on, Thanks!
~Cole7778~
tnx :)
ReplyDelete